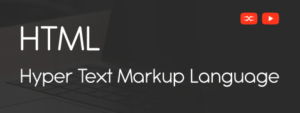
HTML
HTML Div Element
To define a section in an HTML document, <div> tag is used. We use it to group large segments of HTML elements and format them with cascading style sheets. The </div> tag indicates the end of the section.
<!DOCTYPE html>
<html>
<body>
<div>
<p>This is a text inside a div.</p>
</div>
</body>
</html>
Attributes
Some significant characteristics of the < div > tag are as follows:
Align: It specifies the alignment of the div. The possible values are center, right, and left. The default value is left.
<!DOCTYPE html>
<html>
<body>
<div align="right">
<p>Learning Axis</p>
</div>
</body>
</html>
Style: It includes an inline cascading style sheet.
<!DOCTYPE html>
<html>
<body>
<div style="color: red; text-align: center">
<p>Learning Axis</p>
</div>
</body>
</html>
In the above example, the text is in red and centered horizontally.
Uses
We use div elements to structure different sections of a webpage, such as headers, content areas, sidebars, and footers.
<!DOCTYPE html>
<html>
<head>
<style>
.header, .content, .footer {
padding: 20px;
text-align: center;
}
.header {
background-color: #f4b400;
}
.content {
background-color: #0f9d58;
}
.footer {
background-color: #db4437;
}
</style>
</head>
<body>
<div class="header">Header</div>
<div class="content">Content Area</div>
<div class="footer">Footer</div>
</body>
</html>
Let’s see some examples for styling the div elements using CSS.
Flex
The flex property in CSS transforms a container element into a flexible box layout. It allows the arrangement and distribution of its child elements horizontally or vertically.
<!DOCTYPE html>
<html>
<head>
<style>
.outer-div{
display: flex;
justify-content: space-between;
}
.inner-div{
width: 32%;
background-color: blue;
}
</style>
</head>
<body>
<div class="outer-div">
<div class="inner-div">Box-1</div>
<div class="inner-div">Box-2</div>
<div class="inner-div">Box-3</div>
</div>
</body>
</html>
The above example shows a flex container outer-div that evenly spaces three inner-div elements, each occupying 32% of the width and having a blue background.
Grid
In CSS, the grid allows to define the rows and columns, creating a structured layout for organizing elements precisely within the container.
<!DOCTYPE html>
<html>
<head>
<style>
.outer-div{
display: grid;
grid-template-columns: repeat(2, 1fr);
gap: 20px;
}
.inner-div{
background-color: yellow;
}
</style>
</head>
<body>
<div class="outer-div">
<div class="inner-div">Box-1</div>
<div class="inner-div">Box-2</div>
<div class="inner-div">Box-3</div>
<div class="inner-div">Box-4</div>
</div>
</body>
</html>
The above code creates a webpage with a grid layout. The outer div contains four inner div elements arranged in two columns with a 20px gap. Each inner div has a yellow background.