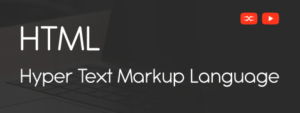
HTML
HTML APIs
API stands for “Application Programming Interface.” It is a set of methods and tools that lets a developer manipulate HTML elements via JavaScript and make them more interactive
What is HTML API for?
HTML API bridges the gap and enables an HTML document to be more responsive and interactive for the end user. It serves the following functions:
- Improving the functionality of the browser.
- Simplifying complex functions and
- Structuring complex codes
The Document Object Model (DOM) is used to access and manipulate documents within HTML. The Accessibility Object Model (AOM) allows developers to modify HTML pages’ accessibility.
<h3 id="heading">HTML API</h3>
<button id="changeTextBtn">Change Text</button>
<script>
// Accessing the DOM to manipulate the HTML element
document.getElementById("changeTextBtn").
addEventListener("click", function() {
// Modifying the content of the HTML element using the API
document.getElementById("heading").
innerText = "You clicked the button!";
});
</script>
HTML Element APIs
To manipulate an HTML document using JavaScript, you will need an object. The DOM program provides the object through which you can manipulate HTML documents easily at any level. Element nodes contain information about HTML attributes which you can manipulate and update.
<p id="message">HTML Element APIs</p>
<button id="updateBtn">Click</button>
<script>
// Access the paragraph element and change its text
document.getElementById("updateBtn").addEventListener
("click", function() {
document.getElementById("message").
textContent = "You clicked the button";
});
</script>
HTML Geolocation API
Geolocation API is used to get information about the user’s current location. However, this information is only possible if the user shares his location while visiting the page.
<button onclick="getLocation()">Get Location</button>
<p id="location"></p>
<script>
function getLocation() {
navigator.geolocation.getCurrentPosition(function(position) {
document.getElementById("location").textContent =
`Lat: ${position.coords.latitude},
Lon: ${position.coords.longitude}`;
});
}
</script>
HTML Drag & Drop API
The Drag-and-Drop API allows users to move items from one place to another. To add this feature, developers need to make the element draggable.
<style>
#dragItem {
width: 50px;
height: 50px;
background-color: lightblue;
text-align: center;
line-height: 50px;
cursor: grab;
}
#dropArea {
width: 100px;
height: 100px;
border: 2px solid black;
margin-top: 10px;
}
</style>
<div id="dropArea"></div>
<div id="dragItem" draggable="true">Drag</div>
<script>
// Allow dragging
document.getElementById("dragItem").addEventListener
("dragstart", function(e) {
e.dataTransfer.setData("text", e.target.id);
});
// Allow dropping
document.getElementById("dropArea").addEventListener
("dragover", function(e) {
e.preventDefault();
});
// Drop the item
document.getElementById("dropArea").addEventListener
("drop", function(e) {
e.preventDefault();
const data = e.dataTransfer.getData("text");
e.target.appendChild(document.getElementById(data));
});
</script>
HTML Web Storage API
This API stores data on a web browser. Conventionally, the data was stored as cookies but is now stored as an HTML web storage API. It can store large amounts of data and is transferable.
<input type="text" id="nameInput" placeholder="Enter your name">
<button onclick="saveName()">Save Name</button>
<button onclick="showName()">Show Name</button>
<p id="display"></p>
<script>
// Save name to localStorage
function saveName() {
const name = document.getElementById("nameInput").value;
localStorage.setItem("username", name);
document.getElementById("nameInput").value = '';
}
// Retrieve and display name from localStorage
function showName() {
const storedName = localStorage.getItem("username");
document.getElementById("display").textContent = storedName ?
`Hello, ${storedName}!` : "No name saved.";
}
</script>
HTML Web Worker API
This HTML API helps developers upload JavaScript smoothly without interrupting the web page’s performance. It runs a JavaScript function in the background, which does not affect any other functionalities on the page.
<p>Count numbers: <output id="result"></output></p>
<button onclick="startWorker()">Start Worker</button>
<button onclick="stopWorker()">Stop Worker</button>
<script>
var w;
function startWorker() {
if (typeof(Worker) !== "undefined") {
if (typeof(w) == "undefined") {
w = new Worker(URL.createObjectURL(new Blob([`
let count = 0;
setInterval(function() {
postMessage(count++);
}, 1000); // Send count every second
`])));
w.onmessage = function(event) {
document.getElementById("result").innerHTML = event.data;
};
}
} else {
document.getElementById("result").innerHTML =
"Sorry, your browser does not support Web Workers...";
}
}
function stopWorker() {
if (w) {
w.terminate();
w = undefined;
}
}
</script>
HTML SSE API
The SSE in HTML SSE API stands for server sent event. The function of HTML SSE API is to get automatic updates from the server. In other words, with this HTML API element, the web page does not need to ask if there are any available updates. The web page gets the update automatically with the server-sent event.
<!-- Client-side (HTML + JavaScript) -->
<div id="time"></div>
<script>
const eventSource = new EventSource('/events');
eventSource.onmessage = function(event) {
document.getElementById('time').innerText = event.data;
};
</script>
<!-- Server-side (Node.js): -->
const express = require('express');
const app = express();
app.get('/events', (req, res) => {
res.setHeader('Content-Type', 'text/event-stream');
res.setHeader('Cache-Control', 'no-cache');
res.setHeader('Connection', 'keep-alive');
setInterval(() => {
const data = new Date().toLocaleTimeString();
res.write(`data: ${data}\n\n`);
}, 1000); // Send the current time every second
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
API consists of pre-built programs or codes developers use to manipulate a document using JavaScript according to project needs.