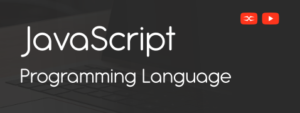
JavaScript
What is JavaScript?
JavaScript is an interpreted language that is utilized to add dynamic content to website pages. Interpreted means that the whole web page is downloaded to the browser and the JavaScript code is executed when an event is triggered. When a code is executed it is interpreted one line at a time. There are various events that will trigger the execution of JavaScript such as a click on a form button or the completion of a web page loading etc.
JavaScript is used to include scrolling text, messages, animations, menus, pop-up windows, etc. JavaScript is directly embedded in HTML.
JavaScript was developed by Sun Microsystems and Communicator. It is an open language. It tends to be used without purchasing. Netscape supports JavaScript and Internet Explorer supports JScript. JScript is a subset of JavaScript.
Running JS
JavaScript is written in an HTML file. A special tag is used to indicate that JavaScript statements have started. The browser executes these statements in different ways than simple HTML tags. It interprets JavaScript statements to generate HTML tags and then displays the result of these tags. The browser uses a built-in JavaScript engine to interpret these statements.
<html>
<head></head>
<body>
<script></script>
</body>
</html>
Aspects
Versatility
Client-Side Scripting: JavaScript is mainly recognized for its ability to operate in the user’s web browser and improve the interaction of websites.
Server-Side Development: JavaScript may be used for server-side scripting using Node.js, which enables programmers to create server-side logic in JavaScript.
Characteristics
Dynamic Content: A webpage’s content and style can be changed dynamically thanks to JavaScript’s ability to manipulate HTML and CSS.
Event Handling: It can react to various user events, including clicks, mouse clicks, and keyboard inputs.
Asynchronous Programming: JavaScript effectively handles asynchronous actions with the help of promises, callbacks, and async/await.
syntax
JavaScript offers support for object-oriented programming paradigms in its syntax.
Loosely Typed: This language is dynamically typed, which offers flexibility but necessitates close consideration of variable types. C-like Syntax: Individuals acquainted with C, C++, or Java may learn it fairly quickly because of its syntax resemblance to C languages.
Development Tools
Developer Tools for Browsers: Developer tools for debugging, profiling, and analyzing JavaScript code are included with most modern browsers.
IDEs and Text Editors: JavaScript programming is supported by several integrated development platforms (WebStorm, Atom) and text editors (VSCode, Sublime Text) that offer capabilities like code completion, syntax highlighting, and debugging.
Script Tag
The < script > tag informs the browser about the start of JavaScript statements. The <script> tag indicates the beginning of scripting code and the </script> tag indicates the end.
Attributes of script tag
Some important attributes of the <script> tag are as follows:
language: Specifies the scripting language used for writing the code. The possible values are JavaScript or VBScript.
src: Specifies the JavaScript source file. The attribute is used if JavaScript is stored in another file with the “.js” extension.
<!DOCTYPE html>
<html lang="en">
<head>
<title><!-- Script Tag Example --></title>
</head>
<body>
<h1>Hello, this is a script tag example!</h1>
<!-- Embedded JavaScript Code -->
<script>
alert('Welcome to the script tag example!');
</script>
<!-- External JavaScript File -->
<script src="js file path"></script>
</body>
</html>