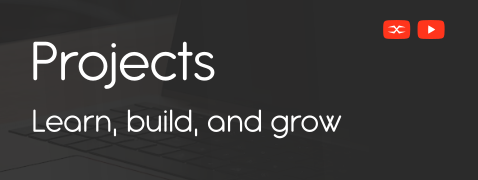
Projects
Web Integrations
Responsive Dropdown Menu
A navigation menu is a fundamental part of any website. In this tutorial, we will build a responsive dropdown menu that works on both desktop and mobile using HTML, CSS, and JavaScript.
Features of this Menu
- Multi-level dropdown support
- Mobile-friendly with a toggle button
- Smooth hover and click-based interactions
HTML
We start with a basic HTML structure for the navigation menu. The menu is generated dynamically using JavaScript, making it easy to modify.
<body>
<nav class="menu-container">
<div class="menu-toggle" id="menuToggle">☰ Menu</div>
<div id="menu"></div>
</nav>
</body>
Following are some Key features of the above HTML.
- <nav>: Wraps the entire navigation menu.
- .menu-container: A wrapper for the menu.
- .menu-toggle: A button that appears in mobile view to show/hide the menu.
- <div id=”menu”>: The empty div where our JavaScript will dynamically generate the menu.
CSS
Now, let’s add styling to make our menu visually appealing and responsive.
/* Reset default styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* Body styling */
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
display: flex;
justify-content: center;
}
/* Navigation container */
.menu-container {
width: 80%;
margin-top: 50px;
}
/* Main menu styles */
#menu ul {
display: flex;
list-style: none;
background-color: #333;
}
#menu li {
position: relative;
}
#menu li a {
display: block;
color: white;
padding: 15px 20px;
text-decoration: none;
}
#menu li a:hover {
background-color: #555;
}
/* Submenu styles */
#menu li ul {
display: none;
position: absolute;
top: 100%;
left: 0;
background-color: #444;
min-width: 180px;
border-radius: 5px;
}
#menu li ul li a {
padding: 12px 20px;
}
#menu li ul li a:hover {
background-color: #666;
}
/* Show submenu on hover (Desktop) */
@media (min-width: 768px) {
#menu li:hover > ul {
display: block;
}
}
/* Multi-level submenus */
#menu li ul li {
position: relative;
}
#menu li ul li ul {
top: 0;
left: 100%;
display: none;
}
/* Mobile Menu */
.menu-toggle {
display: none;
background-color: #333;
color: white;
padding: 15px;
cursor: pointer;
}
/* Mobile menu styling */
@media (max-width: 768px) {
.menu-toggle {
display: block;
}
#menu {
flex-direction: column;
display: none;
}
#menu.active {
display: flex;
}
#menu ul {
flex-direction: column;
}
#menu li a {
padding: 15px;
border-bottom: 1px solid #444;
}
#menu li.active > ul {
display: block;
}
#menu li ul li.active > ul {
display: block;
}
#menu li ul {
position: static;
}
}
In the above CSS, we use the following Key Features.
- The menu bar is styled with a dark background (#333).
- The main menu items are displayed in a horizontal row (display: flex).
- The submenu (#menu li ul) is hidden by default and is shown when the user hovers over a menu item (#menu li:hover > ul).
- On mobile devices, the menu is hidden by default and appears when the toggle button is clicked.
- The @media (max-width: 768px) rule applies mobile-specific styles.
JavaScript
Now, let’s add JavaScript to dynamically generate the menu and handle mobile toggle functionality.
const menuData = [
{
title: "Home",
link: "#"
},
{
title: "About",
link: "#",
submenu: [
{
title: "Company",
link: "#"
},
{
title: "Team",
link: "#",
submenu: [
{ title: "Management", link: "#" },
{
title: "Developers",
link: "#",
submenu: [
{
title: "Frontend",
link: "#",
submenu: [
{ title: "HTML", link: "#" },
{ title: "CSS", link: "#" },
{ title: "JavaScript", link: "#" }
]
},
{ title: "Backend", link: "#" },
]
}
]
}
]
},
{
title: "Services",
link: "#",
submenu: [
{
title: "Web Development",
link: "#",
submenu: [
{ title: "Frontend", link: "#" },
{ title: "Backend", link: "#" }
]
},
{ title: "SEO", link: "#" }
]
},
{
title: "Contact",
link: "#"
}
];
// Function to create the menu
function createMenu(menuItems, depth = 0) {
const ul = document.createElement('ul');
menuItems.forEach(item => {
const li = document.createElement('li');
const a = document.createElement('a');
a.href = item.link;
a.textContent = item.title;
// Handle mobile submenu toggle
if (window.innerWidth <= 768) {
a.style.paddingLeft = `${15 + (depth * 20)}px`;
a.addEventListener('click', (e) => {
if (item.submenu) {
e.preventDefault();
li.classList.toggle('active');
}
});
}
li.appendChild(a);
if (item.submenu) {
const subMenu = createMenu(item.submenu, depth + 1);
li.appendChild(subMenu);
}
ul.appendChild(li);
});
return ul;
}
// Populate Menu
document.getElementById('menu').appendChild(createMenu(menuData));
// Toggle Menu on Mobile
document.getElementById('menuToggle').addEventListener('click',
() => {
document.getElementById('menu').classList.toggle('active');
});
Following are the Key Features we used in JavaScript.
- The menu structure is stored as an array (menuData).
- The createMenu() function recursively generates <ul> and <li> elements.
- Clicking a menu item on mobile devices expands/collapses the submenu (li.classList.toggle (‘active’)).
- The menu toggle button shows/hides the menu when clicked.
Download The Source File
Get immediate access to the original source file with a simple click, and start customizing it to fit your needs.