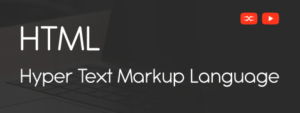
HTML
HTML Classes
HTML class is an attribute that defines one or more class names for any HTML element. CSS and JavaScript use classes to select and manipulate groups of elements with the same class name. The syntax for defining a class is as follows.
<tag class="class-name">Content</tag>
HTML Classes with CSS
The primary use of HTML classes is to apply CSS styles to elements. We can create frequently used styles by defining a class in one or more HTML tags and then referencing it in CSS.
Basic Styling
Let’s start with a simple example. Define the class in HTML to style all buttons with a specific look and apply the styling to the class in CSS.
<!DOCTYPE html>
<html>
<head>
<style>
.btn {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<button class="btn">Click Me</button>
<button class="btn">Submit</button>
</body>
</html>
In the above example, the CSS will apply the same styles to all elements with the btn class, giving buttons a uniform appearance.
Combining Multiple Classes
We can also combine multiple classes to apply different styles to an element. For instance, we have a primary button and a secondary button with slightly different styles.
<!DOCTYPE html>
<html>
<head>
<style>
.btn {
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
.primary {
background-color: #4CAF50;
color: white;
}
.secondary {
background-color: #f1f1f1;
color: black;
}
</style>
</head>
<body>
<button class="btn primary">Primary Button</button>
<button class="btn secondary">Secondary Button</button>
</body>
</html>
In this example, both buttons share the base btn
styles, but each has additional styles specific to their purpose.
HTML Classes with JavaScript
HTML classes are not only for styling. They are also used in adding interactivity to the web pages using JavaScript.
Toggling Classes
In the following example, a JavaScript operation is toggling a class on an element to change its state. We use it for multiple features like dropdown menus, modals, or toggling dark mode.
<!DOCTYPE html>
<html>
<head>
<style>
.highlight {
background-color: yellow;
}
</style>
</head>
<body>
<button id="toggleBtn">Toggle Highlight</button>
<p id="text" class="highlight">This is a paragraph.</p>
<script>
document.getElementById('toggleBtn').addEventListener('click', function () {
document.getElementById('text').classList.toggle('highlight');
});
</script>
</body>
</html>
When we click the button, the highlight class will be toggled on the paragraph, adding or removing the yellow background.
Adding and Removing Classes
Sometimes, we add or remove some classes based on certain conditions. It can be easily achieved using JavaScript.
<!DOCTYPE html>
<html>
<head>
<style>
.highlight {
background-color: yellow;
}
</style>
</head>
<body>
<button id="addClassBtn">Add Class</button>
<button id="removeClassBtn">Remove Class</button>
<p id="text">This is a paragraph.</p>
<script>
document.getElementById('addClassBtn').addEventListener('click', function () {
document.getElementById('text').classList.add('highlight');
});
document.getElementById('removeClassBtn').addEventListener('click', function () {
document.getElementById('text').classList.remove('highlight');
});
</script>
</body>
</html>
In this example, clicking the button (Add Class) will add the highlight class to the paragraph, and clicking the button (Remove Class) will remove it.
Remember, the key to using the classes effectively is to name them logically and consistently, ensuring styles and scripts can be easily understood and managed.