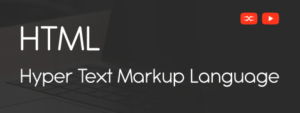
HTML
HTML Forms Attributes
The HTML form attribute defines how the user’s input is processed and managed. There are different types of HTML form attributes. These include:
<form action="/contact-us/" method="POST" autocomplete="off">
<input type="text" name="username" placeholder="Username">
<input type="email" name="email" required>
<input type="submit" value="Submit">
</form>
The Action Attribute
This attribute defines an action that needs to be performed when a user submits an HTML form.
<form action="/contact-us/">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<input type="submit" value="Submit">
</form>
action=”/submit-form”: When the form is submitted, the action attribute will send data to the specified URL (e.g., /submit-form).
The Target Attribute
This attribute is related to the response that occurs when you hit the submit button to submit the form.
<form action="/contact-us/" target="_blank">
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" value="Submit">
</form>
target= “_blank”: The form will be submitted, and the response will open in a new tab.
target= “_self”: (Default) The response will load in the same tab as the current page.
target= “_parent”: If the page is inside an iframe, the response will load in the parent frame.
target= “_top”: The response will load in the whole body of the window.
target= “framename”: The response will load in a specific iframe or frame.
The Method Attribute
This attribute describes the HTTP method that will be used while submitting the form. The data can be sent in the form of URL variables or as HTTP post transaction.
<form action="/contact-us/" method="POST">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<input type="submit" value="Submit">
</form>
method= “POST”: The form data is sent as part of the request body, which is more secure and suitable for handling sensitive information or large amounts of data.
method= “GET”: Form data is added to the URL as query parameters. This method is helpful for simple forms where the data can be seen in the URL, but it’s less secure and has a data length limitation.
The Autocomplete Attribute
This attribute defines whether the autocomplete feature in a form is ON or OFF. When it’s ON, the attribute automatically fills in the values based on the previous action.
<form action="/contact-us/" method="POST" autocomplete="on">
<label for="name">Name:</label>
<input type="text" id="name" name="name" autocomplete="name">
<label for="email">Email:</label>
<input type="email" id="email" name="email" autocomplete="email">
<input type="submit" value="Submit">
</form>
autocomplete= “on”: (Default) The browser will suggest and auto-fill form values based on the user’s previous entries.
autocomplete= “off”: Disables the browser’s autocomplete feature, preventing it from suggesting or auto-filling form values.
autocomplete= “name” & autocomplete= “email”: These attributes on individual input fields guide the browser to use specific data types for autocomplete, like names or emails.
The Novalidate Attribute
It is a boolean attribute that saves the details but does not validate the input when the form is submitted.
<form action="/contact-us/" method="POST" novalidate>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="18" required>
<input type="submit" value="Submit">
</form>
novalidate: When this attribute is added to the <form> tag, the browser will bypass its default form validation. For example, the form can still be submitted even if the email field is not correctly formatted or the age field is below the required minimum.