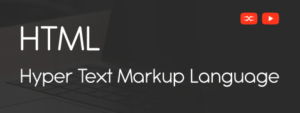
HTML
HTML Input Attributes
Various HTML attributes are used to describe HTML input elements. For example:
The Value Attribute
It defines the initial values of an input field.
<form>
<!-- Text Input: Pre-filled with "Axis" as the default value -->
<input type="text" id="username" name="username" value="Axis">
<!-- Radio Button: "Male" is selected by default -->
<input type="radio" name="gender" value="male" checked> Male
<input type="radio" name="gender" value="female"> Female
<!-- Submit Button: Displays "Send" as the button text -->
<input type="submit" value="Send">
<!-- Range Input: Starts at value 50 by default -->
<input type="range" id="volume" name="volume"
min="0" max="100" value="50">
</form>
The Read Only Attribute
It defines that a specific field is read-only. This field can not be edited or modified.
<form>
<!-- Text Input with Readonly Attribute -->
<input type="text" name="username" value="Axis" readonly>
<!-- Textarea with Readonly Attribute -->
<textarea id="bio" name="bio" readonly>
This text cannot be changed.
</textarea>
</form>
The Disabled Attribute
It is used to turn off a specific field in the HTML form. It is unusable and unclickable.
<form>
<!-- Disabled Text Input: Non-editable and unselectable -->
<input type="text" name="username" value="Axis" disabled>
<!-- Disabled Textarea: Non-editable and unselectable -->
<textarea id="bio" name="bio" disabled>
This text cannot be changed or selected.
</textarea>
<!-- Disabled Submit Button: Can't be clicked -->
<input type="submit" value="Submit" disabled>
</form>
The Size Attribute
This input attribute is used to define the width of a field.
<form>
<!-- Text Input with Size Attribute -->
<input type="text" id="username" name="username" size="20">
<!-- Select with Size Attribute -->
<select id="cars" name="cars" size="3">
<option value="toyota">Volvo</option>
<option value="honda">Saab</option>
</select>
</form>
The Max length Attribute
This HTML input attribute specifies the maximum length or number of characters that can be added to an input field.
<form>
<!-- Text Input with Maxlength Attribute -->
<input type="text" id="username" name="username" maxlength="10">
<!-- Textarea with Maxlength Attribute -->
<textarea id="bio" name="bio" maxlength="50"></textarea>
</form>
The Min and Max Attributes
This input attribute specifies the minimum and maximum number of characters or values that can be entered in an input field.
<form>
<!-- Number Input with Min and Max Attributes -->
<input type="number" id="age" name="age" min="18" max="65">
<!-- Date Input with Min and Max Attributes -->
<input type="date" name="start" min="2023-01-01" max="2024-12-31">
<!-- Range Input with Min and Max Attributes -->
<input type="range name="volume" min="0" max="100" value="50">
</form>
The Multiple Attribute
The multiple attribute allows a user to add more than one response in a specific input field. It works best with emails and files.
<form>
<!-- File Input with Multiple Attribute -->
<input type="file" id="files" name="files" multiple>
<!-- Select Element with Multiple Attribute -->
<select id="fruits" name="fruits" multiple>
<option value="apple">Apple</option>
<option value="orange">Orange</option>
</select>
</form>
The Pattern Attribute
This input attribute checks the user’s response when the HTML form is submitted with a regular expression.
<form>
<!-- Text Input with Pattern Attribute -->
<input type="text" id="phone" name="phone"
pattern="\d{3}[-]\d{3}[-]\d{4}"
placeholder="123-456-7890" >
<!-- Email Input with Pattern Attribute -->
<input type="email" id="email" name="email"
pattern="[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}"
placeholder="example@domain.com">
</form>
The Placeholder Attribute
The placeholder attribute adds a hint in the input field to let the user know the expected value for that specific field.
<form>
<!-- Text Input with Placeholder Attribute -->
<input type="text" name="username"
placeholder="Enter your username">
</form>
The Required Attribute
It defines those fields that must be filled, and the form should not submitted without filling these out.
<form>
<!-- Required Text Input -->
<input type="text" id="username" name="username" required>
<!-- Required Email Input -->
<input type="email" id="email" name="email" required>
<!-- Select Input with Required Attribute -->
<select id="country" name="country" required>
<option value="">Select your country</option>
<option value="usa">United States</option>
</select>
</form>
The Step Attribute
The list attribute is used to add a pre-defined list of options to an input field. It contains limited options from which users can select their response.
<form>
<!-- Number Input with Step Attribute -->
<input type="number" id="age" name="age" min="0" step="5">
<!-- Time Input with Step Attribute -->
<input type="time" id="time" name="time" step="900">
</form>
The Autofocus Attribute
It is used to specify a particular field that should be focused automatically when the user loads a page.
<form>
<!-- Text Input with Autofocus Attribute -->
<input type="text" id="username" name="username" autofocus>
<!-- Password Input without Autofocus -->
<input type="password" id="password" name="password">
</form>
The List Attribute
To add a pre-defined list of options in an input field, the list attribute is used. It contains limited options from which the users can select their response.
<form>
<!-- Text Input with List Attribute -->
<input type="text" id="color" name="color" list="colors">
<!-- Datalist with Predefined Options -->
<datalist id="colors">
<option value="Red">
<option value="Green">
</datalist>
</form>
Input Type Image
This HTML input type allows to define and use an image as a submit button for the form.
<form>
<!-- Image Submit Button -->
<input type="image" src="#" alt="Submit">
</form>
The Height and Width Attribute
This input attribute is used to specify the height and width of an input image.
<form>
<!-- Image Submit Button with Height and Width Attributes -->
<input type="image" src="#" alt="Submit" width="50" height="20">
</form>
The Autocomplete Attribute
It defines whether an HTML form or a specific field should have an autocomplete option ON or OFF. This attribute allows the browser to predict the user’s response.
<form>
<!-- Text Input with Autocomplete Enabled -->
<input type="text" name="username" autocomplete="on">
<!-- Password Input with Autocomplete Disabled -->
<input type="password" name="password" autocomplete="off">
</form>