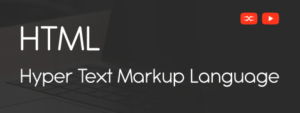
HTML
HTML Styles
HTML styles allow designers to add color, shape, and texture to the web page.
We can add CSS directly to an HTML document using the <style> tag or a link to an external CSS file. We can also apply styles inline using the style attribute.
Inline Styles
Inline styles can be applied directly to individual HTML elements using the style attribute. This styling is known as inline CSS.
<!DOCTYPE html>
<html>
<body>
<h3 style="color: blue;">Welcome to my Website</h3>
<p style="font-size: 16px;">This is an example</p>
</body>
</html>
In the above example, CSS styles such as color and font size are applied directly to the <h3> and <p> elements using the style attribute.
While inline CSS can be convenient for small-scale projects or when specific styles are required for individual elements, it is generally not recommended for larger projects due to its lack of scalability and maintainability.
Internal Styles
Internal styles can be added directly in the HTML file, typically within the <style> tags in the <head> section of the document. This approach allows you to define styles specific to that HTML document without creating a separate CSS file.
This styling method is known as Internal CSS.
<!DOCTYPE html>
<html lang="en">
<head>
<style>
body {
font-family: Arial, sans-serif;
}
h3 {
color: blue;
}
p {
font-size: 18px;
}
</style>
</head>
<body>
<h3>Learning Axis</h3>
<p>This is a paragraph with some text.</p>
</body>
</html>
In the above example, all the styles are in the <style> tag. These styles will only apply to the elements within this specific HTML document. Internal CSS can be best for small projects or when you want to keep all the code for a particular webpage in one file.
External Styles
External styling is a way of styling HTML documents where CSS code is stored in a separate file with a .css extension and linked to the HTML document using the <link> element. This keeps CSS separate from the HTML code, making it easier to maintain and manage, especially in big projects or when multiple HTML files have the same style.
This styling is called External CSS
Here’s an example of how external CSS.
Create a separate CSS file (e.g., styles.css) and define your styles.
body {
font-family: Arial, sans-serif;
}
h3 {
color: blue;
}
p {
font-size: 18px;
}
Link this CSS file to the HTML document using the <link> tag.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h3>This is a heading</h3>
<p>This is a paragraph with some text.</p>
</body>
</html>
In the above example, we use external CSS and link to the HTML document using the <link> tag with the href attribute. Any styles defined in styles.css will apply to the HTML elements in the linked HTML document.
External CSS offers several advantages, including easier maintenance, better organization, and the ability to apply the same styles across multiple HTML documents. It also allows for better collaboration since developers can work on the CSS file separately from the HTML content.