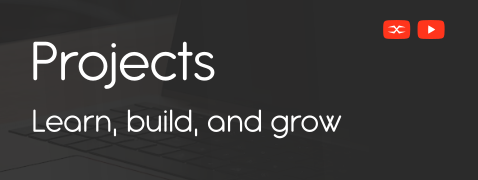
Projects
Web Integrations
Responsive Header
A responsive header is an essential component of modern web design. It ensures seamless navigation across various screen sizes, providing a user-friendly experience on desktops, tablets, and mobile devices. In this guide, we will explore the structure, styling, and functionality of a responsive header created using HTML, CSS, and JavaScript.
Structure of the Header
The header consists of a navigation bar that includes:
A logo positioned on the left side.
A menu with links to different sections of the website.
A hamburger icon that appears on smaller screens, allowing users to toggle the navigation menu.
HTML
The HTML structure is simple yet effective. The <nav> element contains:
- A logo wrapped inside an anchor tag.
- A menu list inside a div with an ID of navLinks.
- A hamburger icon (using <i> and <div> elements) for mobile responsiveness.
<nav class="nav-a">
<a href="#" class="logo">LOGO</a>
<div class="nav-links-a" id="navLinks">
<i class="fa fa-close" onclick="hideMenu()">X</i>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</div>
<i class="fa fa-menu" onclick="showMenu()">
<div class="a"></div>
<div class="a"></div>
<div class="a"></div>
</i>
</nav>
Styling the Header with CSS
The CSS styles ensure a clean and responsive design:
- The navigation bar is styled with display: flex; to align elements properly.
- The menu items use inline-block display for a horizontal layout.
- A hover effect is added to highlight menu items when the cursor moves over them.
* {
margin: 0;
padding: 0;
font-family: 'poppins', sans-serif;
}
.nav-a {
height: 6rem;
display: flex;
justify-content: space-between;
align-items: center;
border-bottom: 2px solid #eee;
padding-left: 2%;
padding-right: 2%;
}
.nav-a .logo {
text-decoration: none;
font-size: 40px;
font-weight: 700;
color: blueviolet;
}
.nav-links-a {
flex: 1;
text-align: right;
}
.nav-links-a ul li {
list-style: none;
display: inline-block;
padding: 8px 12px;
position: relative;
}
.nav-links-a ul li a {
color: #555555;
text-decoration: none;
font-size: 18px;
}
.nav-links-a ul li ::after {
content: '';
width: 0%;
height: 2px;
background-color: blueviolet;
display: block;
margin: auto;
transition: 0.5s;
}
.nav-links-a ul li:hover ::after {
width: 100%;
}
.fa {
display: none;
}
.a {
height: 3px;
width: 25px;
background-color: blueviolet;
margin-top: 4px;
}
Styling the Hamburger Menu with CSS
To ensure the hamburger menu is properly displayed on smaller screens, we apply specific styles:
- The .nav-links-a is hidden by default and positioned off-screen.
- The .fa icon is set to display: block; when the screen width is below 600px.
- A smooth transition effect is added to slide the menu in and out.
@media screen and (max-width: 600px) {
.nav-a {
height: 5rem;
}
.text-box h1 {
font-size: 20px;
}
.nav-links-a ul li {
display: block;
}
.nav-links-a ul li a {
color: #eee;
}
.nav-links-a {
position: fixed;
background-color: blueviolet;
height: 100vh;
width: 200px;
top: 0;
right: -200px;
text-align: left;
z-index: 2;
transition: 1s;
}
.nav-a .fa {
display: block;
color: #eee;
margin: 5px;
font-size: 22px;
cursor: pointer;
}
.fa-close {
padding-left: 10px;
padding-top: 10px;
}
.nav-links-a ul {
padding: 30px;
}
}
Adding JavaScript Functionality
The JavaScript code enables the functionality of the hamburger menu:
When the menu icon is clicked, the menu slides in.
When the close icon is clicked, the menu slides out.
let navLinks = document.getElementById("navLinks");
function showMenu() {
navLinks.style.right = "0";
}
function hideMenu() {
navLinks.style.right = "-200px";
}
This responsive header improves website navigation, providing a seamless experience across different devices. By combining HTML, CSS, and JavaScript, we ensure both functionality and aesthetics. You can modify this design to match your website’s branding and enhance user engagement.
Download The Source File
Get immediate access to the original source file with a simple click, and start customizing it to fit your needs.