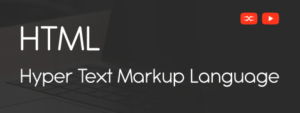
HTML
HTML Responsive
HTML responsive design ensures a web page adapts to different screen sizes seamlessly and looks good on all electronic devices. It offers users an optimal view of the web pages.
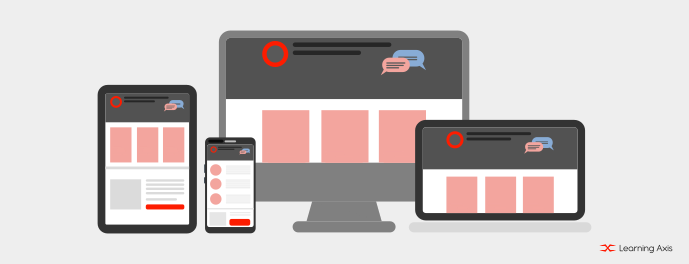
How HTML Responsive Web Design Works?
Users can access web pages using devices like laptops, tablets, and smartphones. HTML responsive design adjusts web pages according to the user’s device. In short, a web page will fit the user’s screen seamlessly, offering a user-friendly view of the page.
<html>
<head>
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
</head>
<body>
<!-- Body Content -->
</body>
</html>
Moreover, it can only use HTML and CSS to adjust, resize, shrink, and expand the content according to screen size.
Steps to Create HTML Responsive Design
Responsive web design offers optimal views to all users and maintains the functionality and aesthetics of a webpage on all platforms and devices. You can make a web page responsive in multiple ways. Some of them are:
Add a viewport meta tag.
<head>
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
</head>
By including responsive typography.
<html>
<head>
<style>
body { font-size: 1rem; }
/* Default size */
@media (min-width: 768px) {
body { font-size: 1.2rem; }
/* Larger size for tablets and above */
}
@media (min-width: 1024px) {
body { font-size: 1.5rem; }
/* More larger for desktops */
}
</style>
</head>
<body>
<h3>Responsive Typography</h3>
<p>This text size changes based on the width of the screen.</p>
</body>
</html>
Adding picture tags to the image also renders the page size on all devices.
<html>
<head>
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
</head>
<body>
<h3>Responsive Images</h3>
<picture>
<source media="(min-width: 800px)" srcset="#">
<source media="(min-width: 500px)" srcset="#">
<img src="#" alt="A beautiful scenery">
</picture>
<p>The image above changes depending
on the device's screen size.</p>
</body>
</html>
A CCS technique, media query can make webpages fit different browser sizes.
<html>
<head>
<style>
.box {
width: 100px;
height: 100px;
background-color: red;
}
@media (min-width: 600px) {
.box {
background-color: blue;
}
}
@media (min-width: 900px) {
.box {
background-color: green;
}
}
</style>
</head>
<body>
<div class="box"></div>
<p>The above box changes color based
on the width of the browser window.</p>
</body>
</html>
Use flexible layout techniques instead of a fixed grid system.
<html>
<head>
<style>
.container {
display: flex;
flex-wrap: wrap;
}
.item {
flex: 1 1 200px;
margin: 10px;
background-color: lightgrey;
padding: 20px;
text-align: center;
}
</style>
</head>
<body>
<div class="container">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<div class="item">Item 4</div>
</div>
<p>The items above rearrange themselves
based on the screen size.</p>
</body>
</html>